One of the tedious chores with mobile apps are the screenshots for Play Store and AppStore, especially because this is the only way how you can showcase the app to potential uses. Also as app grew, it may get even more time consuming since the number of screenshots, supported languages, pre-configurations and features increases over time.
As of now, HabitChallenge app has 90 image files representing permutations of presented screens, languages, devices and supported systems. I really DO NOT want to take all of them manually!!
Here is how I’m doing this right now in fully automated way and can get all of the 90 screenshots after each release in about 8 minutes!
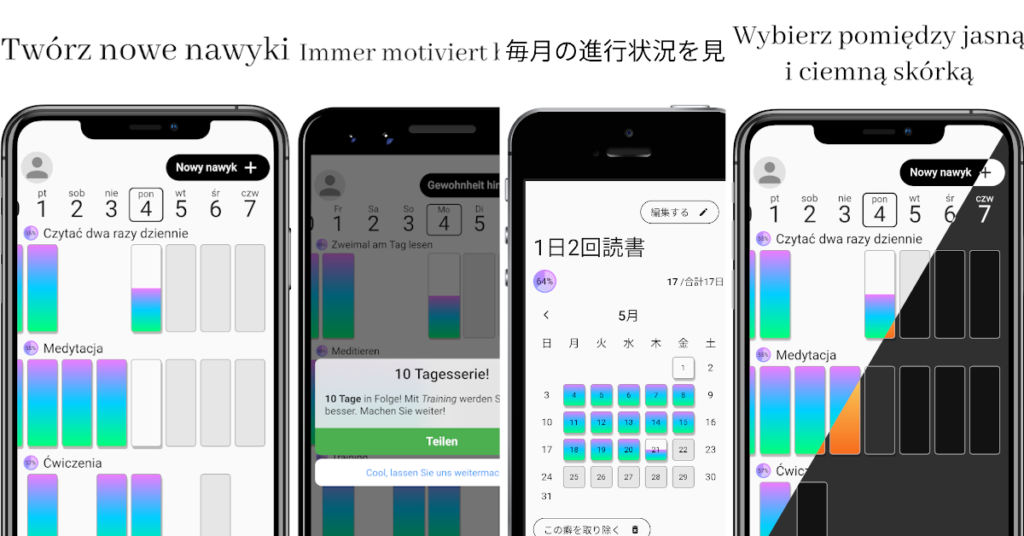
HabitChallenge is written in Flutter right now, and since Flutter controls every single pixel on the screen, has an awesome google_fonts library… and device_frame… and flutter_driver. We can create whole screenshot using dart and flutter!! Without the need for any graphical processing in Photoshop or Gimp! We can upload them straight to the store using fastlane!
OK, let’s stop bragging and dive into technical details!
What are the main ingredients?
- canvas – each store defines different sizes for app screenshots, this will limit the overall size of the final image, also this is where background colour or image can be set,
- header text – about 1/5 of the image height is reserved for the localised header text,
- device frame,
- featured app screen – localised app screen with example data.
0. The Emulator
Actually, before we even start with a first of line of code, we should think from where we’ll get the images. The first thought is from the phone emulator, as we always work with phone emulators, right?
Not quite so. Yes, we’ll create a screenshot for the phone listing in the store, but we also need to remember that each device (and emulator) have different screen resolution and density. Plus we probably don’t want to be bothering about screen safe areas, status and navigation bar. If we use emulator that have the same screen size as our screenshot, we’ll end up with screenshots slightly shorter.
The solution here is to create a new type of device that would have enough screen real estate to fit desired screenshot and not crop it vertically nor horizontally.
Another approach to solving this issue, may be using Flutter web or desktop, but it was easier for me to create huge tablet emulator than explore new Flutter environments.
I’ve created new (TV) android emulator with screen size of 25″ and resolution of 3000x4500px. This beast can easily fit all of the screenshots I need.
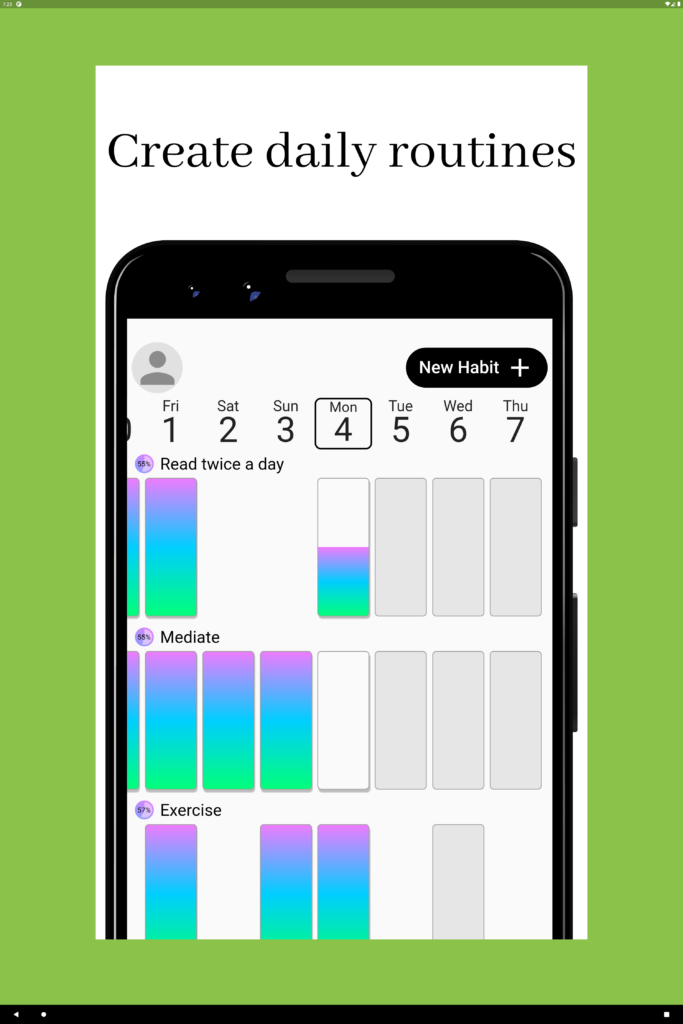
Now, we can look through code samples!
1. The Canvas
Here is the main part of our screenshot, the canvas! It is doing a bit more then just adding a white background.
First of all (1), it is setting the stage of our green screen and center everything inside of it.
Secondly (2), it is setting proper width and height for the real canvas. It is important to note, that we need to divide required screenshot size (in pixels) by the device pixel ratio so that resulting image actually have the width and height we expect. Here we also set the background colour and clip the overflowing edge. TBH, we can skip the clipping, since we’ll crop the whole image using dart’s image library later on.
Next (3), we’re dealing with the localisation of the header text. Since we want the header text to match application language, we need to create Localizations widget and provide it with the configuration. Then, create ScreanshotHeader that is also responsible for the font size and text presentation.
Finally (4), we add the device it self with an embedded screen.
Continue reading